This post includes a compilation of the common concepts regarding the Spring framework. Some noteworthy points:
- Dispatcher Servlet
- Inversion of Control & Dependency Injection (IoC & DI)
- Scope of Beans
Dispatcher Servlet
Spring MVC is designed around the front controller pattern where a central Servlet
, the DispatcherServlet
provides a shared algorithm for request processing, while actual work is performed by configurable delegate components.
This model is flexible and supports diverse workflows.
The DispatcherServlet
needs to be declared and mapped according to the Servlet specification in web.xml
. In turn, the DispatcherServlet
uses Spring configuration to discover the delegate components it needs for request mapping, view resolution, exception handling.
DispatcherServlet
expects a WebApplicationContext
for its own configuration.
WebApplicationContext
has a link to the ServletContext
and the Servlet
with which it is associated. It is also bound to the ServletContext
such that applications can use static methods on RequestContextUtils
to look up the WebApplicationContext
if they need access to it.
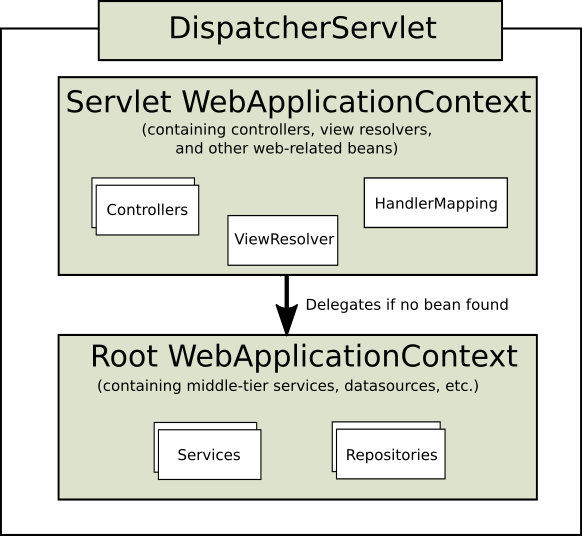
The DispatcherServlet
processes requests as follows:
- The
WebApplicationContext
is searched for and bound in the request as an attribute that the controller and other elements in the process can use. It is bound by default under theDispatcherServlet.WEB_APPLICATION_CONTEXT_ATTRIBUTE
key. - The locale resolver is bound to the request to let elements in the process resolve the locale to use when processing the request (rendering the view, preparing data, and so on). If you do not need locale resolving, you do not need the locale resolver.
- The theme resolver is bound to the request to let elements such as views determine which theme to use. If you do not use themes, you can ignore it.
- If you specify a multipart file resolver, the request is wrapped in a
MultipartHttpServletRequest
for further processing by other elements in the process. - An appropriate handler is searched for. If a handler is found, the execution chain associated with the handler (preprocessors, postprocessors, and controllers) is executed in order to prepare a model or rendering. Alternatively, for annotated controllers, the response can be rendered (within the
HandlerAdapter
) instead of returning a view. - If a model is returned, the view is rendered. If no model is returned (e.g. request interception), no view is rendered, because the request could already have been fulfilled.
The HandlerExceptionResolver
beans declared in the WebApplicationContext
are used to resolve exceptions thrown during request processing.
Connection Pool
A cache of database connections maintained, so that the connections can be reused when future requests to the database are required.
Dependency Injection Related
@Component & @Bean
- @Component: For component scanning and autowiring. Spring auto-detect and auto-configure beans using classpath scanning, implicit one-to-one mapping between the annotated class and the bean (i.e. one bean per class).
- @Bean + @Configuration: explicitly declare a single bean, rather than letting Spring do it automatically. The @Bean annotation returns an object that spring should register as a bean in the Spring application context.
Constructor-Based Dependency Injection
classes with a single constructor can omit the
@Autowired
annotationconstructor-based injection can be leveraged in
@Configuration
annotated classese.g.
1
2
3
4
5
6
7
8
9
10
public class Car {
// Constructor Injection
public Car(Engine engine, Transmission transmission) {
this.engine = engine;
this.transmission = transmission;
}
}
Scope of Beans (6)
前面两个最重要 什么情况下用singleton,什么情况下用 prototype
Six Types:
- Singleton
- Prototype: a single bean definition to any number of object instances
- Used in STATEFUL services, creates new instance for each use case.
- Thread-safe
- Request
- Session
- Application
- Web socket
- Singleton: a single bean definition to a single object instance per Spring IoC container (REST 用的大多数是 Singleton)
- How to implement a singleton pattern?
- private constructor, restrict other classes
- private static singleton variable
- another public static (another var, returns the instance of the var)
The most popular approach is to implement a Singleton by creating a regular class and making sure it has:
- A private constructor
- A static field containing its only instance
- A static factory method for obtaining the instance
Use synchronized for protection